This first edition was written for Lua 5.0. While still largely relevant for later versions, there are some differences.
The fourth edition targets Lua 5.3 and is available at Amazon and other bookstores.
By buying the book, you also help to support the Lua project.
For when you forget or don't know how to take advantage of an eval statement or spawn a shell in a specific language or escape some common programs. Simple inline execution of commands, without semicolons. Lua is dynamically typed based off of the data stored there- This is a string and it can be surrounded by ' or 'name = 'Derek'- Another way to print to the screen- Escape Sequences: n b t ' '- Get the string size by proceeding it with a # io.write ('Size of string ', # name, 'n'). I’ve made a couple of cheat sheets on the topic of Roblox game development, specifically Lua coding using Roblox’s API. Here’s some links to my work! Roblox General Scripting This is a general reference for scripting in Lua on Roblox. This cheat sheet is intended for beginner scripters. Access this cheat sheet here. Roblox Gamepad Continue reading 'Cheat Sheets'.
Programming in Lua |
Part I. The LanguageChapter 3. Expressions |
How To Code In Lua
3.6 – Table Constructors
Constructors are expressions that create andinitialize tables.They are a distinctive feature of Luaand one of its most useful and versatile mechanisms.
The simplest constructor is the empty constructor,{}
, which creates an empty table;we saw it before.Constructors also initialize arrays(called also sequences or lists).For instance, the statementwill initialize days[1]
with the string 'Sunday'
(the first element has always index 1, not 0),days[2]
with 'Monday'
,and so on:
Constructors do not need to use only constant expressions.We can use any kind of expression for the value of each element.For instance, we can build a short sine table as

To initialize a table to be used as a record,Lua offers the following syntax:which is equivalent to
No matter what constructor we use to create a table,we can always add and remove other fields of any type to it:That is, all tables are created equal;constructors only affect their initialization.


Every time Lua evaluates a constructor,it creates and initializes a new table.Consequently, we can use tables to implement linked lists:This code reads lines from the standard inputand stores them in a linked list, in reverse order.Each node in the list is a table with two fields:value
, with the line contents,and next
, with a reference to the next node.The following code prints the list contents:(Because we implemented our list as a stack,the lines will be printed in reverse order.)Although instructive,we hardly use the above implementation in real Lua programs;lists are better implemented as arrays,as we will see in Chapter 11.
Lua Code List
We can mix record-style and list-styleinitializations in the same constructor:The above example also illustrates how we can nest constructorsto represent more complex data structures.Each of the elements polyline[1]
, ..., polyline[4]
is a table representing a record:
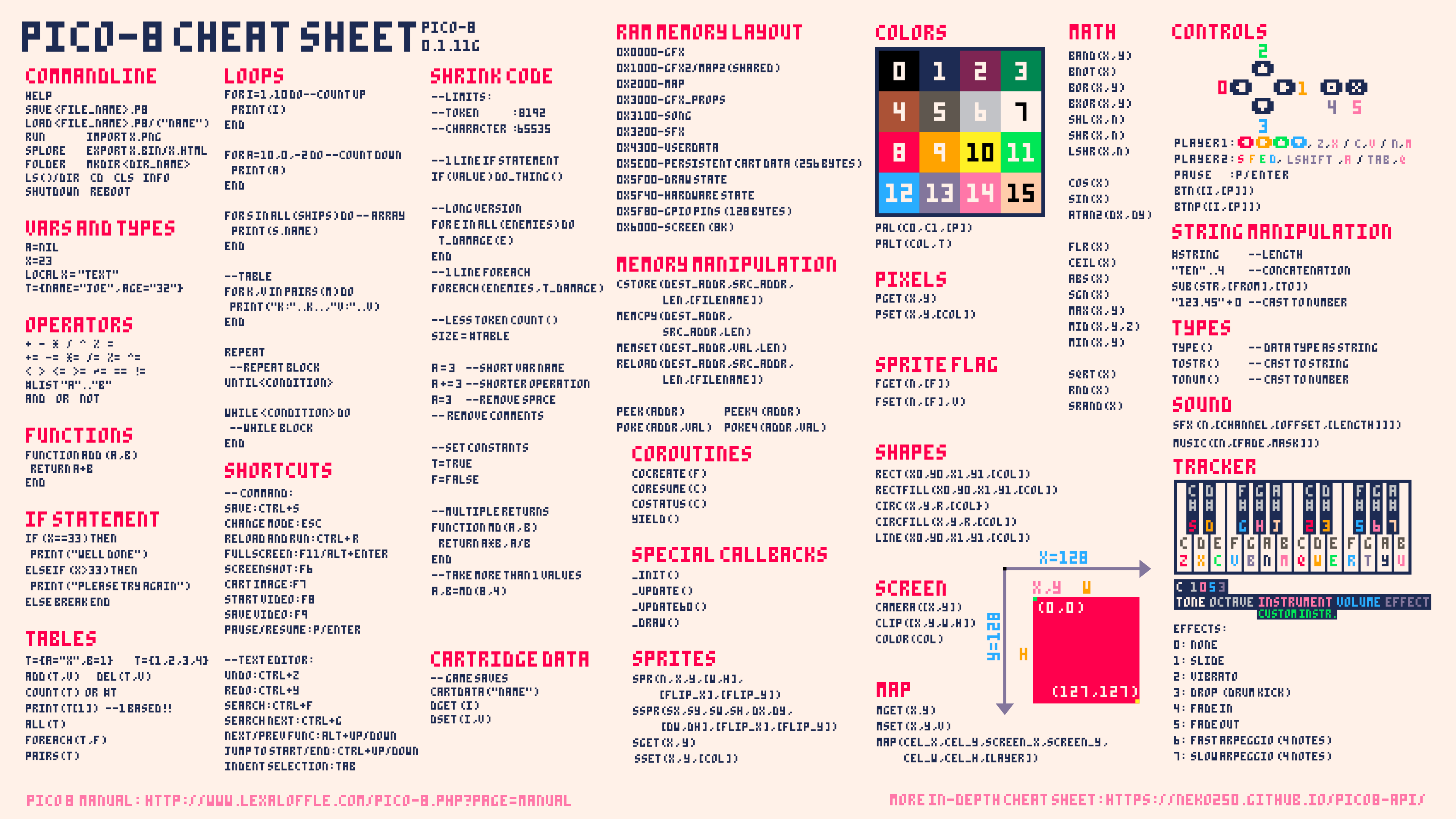
Those two constructor forms have their limitations.For instance,you cannot initialize fields with negative indices,or with string indices that are not proper identifiers.For such needs, there is another, more general, format.In this format,we explicitly write the index to be initialized as an expression,between square brackets:That syntax is more cumbersome, but more flexible too:Both the list-style and the record-style forms are specialcases of this more general one.The constructoris equivalent toand the constructoris equivalent to
For those that really want their arrays starting at 0,it is not difficult to write the following:Now, the first value, 'Sunday'
, is at index 0.That zero does not affect the other fields,but 'Monday'
naturally goes to index 1,because it is the first list value in the constructor;the other values follow it.Despite this facility,I do not recommend the use of arrays starting at 0 in Lua.Remember that most functions assume thatarrays start at index 1,and therefore will not handle such arrays correctly.
Lua Programming Language
You can always put a comma after the last entry.These trailing commas are optional, but are always valid:Such flexibility makes it easier to write programs that generate Lua tables,because they do not need to handle the last element as a special case.
Lua Api Cheat Sheet
Finally, you can always use a semicolon instead of a comma in a constructor.We usually reserve semicolons to delimitdifferent sections in a constructor,for instance to separate its list part from its record part:
List Of Lua Commands
Copyright © 2003–2004 Roberto Ierusalimschy. All rights reserved. |
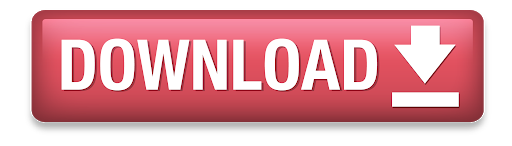